Introduction to CloudFormation StackSets
This page covers AWS StackSets.
AWS CloudFormation StackSets extends the capability of stacks by enabling you to create, update, or delete stacks across multiple accounts and AWS Regions with a single operation. Using an administrator account, you define and manage an AWS CloudFormation template, and use the template as the basis for provisioning stacks into selected target accounts across specified AWS Regions.
Working with AWS CloudFormation StackSets
In this introduction to StackSets, we will use StackSets to deploy resources to two regions of our own account.
Environment
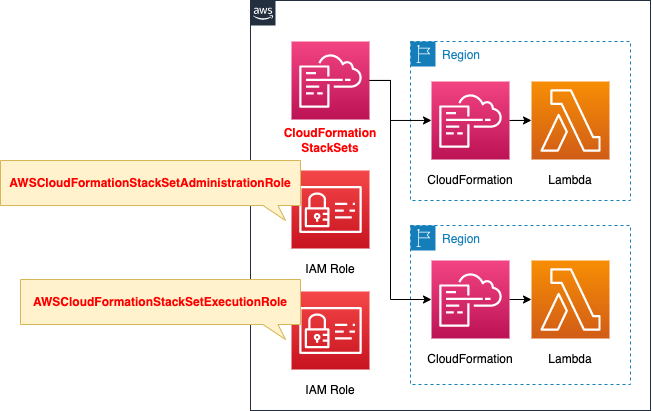
Use CloudFormation StackSets to create CloudFormation stacks for the two regions of your account.
In the stack, create a Lambda function.
Two IAM roles are also created for use in running StackSets.
CloudFormation template files
The above configuration is built with CloudFormation.
The CloudFormation templates are placed at the following URL
https://github.com/awstut-an-r/awstut-fa/tree/main/135
Explanation of key points of template files
StackSets
Resources:
StackSet:
Type: AWS::CloudFormation::StackSet
Properties:
AdministrationRoleARN: !GetAtt AdministrationRole.Arn
Capabilities:
- CAPABILITY_NAMED_IAM
Parameters:
- ParameterKey: Prefix
ParameterValue: !Ref Prefix
- ParameterKey: Handler
ParameterValue: !Ref LambdaHandler
- ParameterKey: Memory
ParameterValue: !Ref LambdaMemory
- ParameterKey: Runtime
ParameterValue: !Ref LambdaRuntime
PermissionModel: SELF_MANAGED
StackInstancesGroup:
- DeploymentTargets:
Accounts:
- !Ref AWS::AccountId
Regions:
- !Ref AWS::Region
- DeploymentTargets:
Accounts:
- ap-northeast-1
ParameterOverrides:
- ParameterKey: Memory
ParameterValue: 256
Regions:
- us-east-1
StackSetName: !Ref Prefix
TemplateURL: !Ref TemplateURL
Code language: YAML (yaml)
We will cover the key points in creating StackSets.
The Parameters property allows you to set parameters for the stack created by StackSets.
In this case, we will create a Lambda function in each region, so we specify the runtime environment and the amount of memory.
The PermissionModel property is a parameter related to access permissions.
You can create stack sets using either self-managed permissions or service-managed permissions.
With self-managed permissions, you create the IAM roles required by StackSets to deploy across accounts and Regions. These roles are necessary to establish a trusted relationship between the account you’re administering the stack set from and the account you’re deploying stack instances to. Using this permissions model, StackSets can deploy to any AWS account in which you have permissions to create an IAM role.
Permission models for stack sets
In this case, “SELF_MANAGED” is specified to select the self-managed type.
The Parameters property allows you to specify parameters to be passed to the generated stack.
In this case, we will set the following parameters for the Lambda function.
- Handler (function to be executed by Lambda): index.lambda_handler
- Memory (amount of Lambda memory): 128
- Runtime (Lambda runtime environment): python3.8
- Prefix (Lambda function name prefix): fa-135
The StackInstancesGroup property is a configuration item related to stack instances.
A stack instance is a reference to a stack in a target account within a Region.
Stack instances
In other words, this stack instance is used to set which region of whose account the stack will be created.
In this case, we will generate stacks in two regions (ap-northeast-1, us-east-1) for our account.
So specify your account ID in the DeploymentTargets property and both regions in the Regions property.
For the stack to be placed on us-east-1, we will consider changing the amount of memory.
In this configuration, the default value is passed as “128” set in the Parameters property mentioned above.
To change this value, the ParameterOverrides property can be used.
This time we will change it to “256”.
IAM Roles
The key to creating self-managed StackSets is the IAM role.
Create two IAM roles.
IAM role for the administrator account
Resources:
AdministrationRole:
Type: AWS::IAM::Role
Properties:
RoleName: AWSCloudFormationStackSetAdministrationRole
AssumeRolePolicyDocument:
Version: 2012-10-17
Statement:
- Effect: Allow
Principal:
Service: cloudformation.amazonaws.com
Action:
- sts:AssumeRole
Path: /
Policies:
- PolicyName: AssumeRole-AWSCloudFormationStackSetExecutionRole
PolicyDocument:
Version: 2012-10-17
Statement:
- Effect: Allow
Action:
- sts:AssumeRole
Resource:
- arn:*:iam::*:role/AWSCloudFormationStackSetExecutionRole
Code language: YAML (yaml)
The first is the IAM role for the administrator account.
In the administrator account, create an IAM role named AWSCloudFormationStackSetAdministrationRole. The role must have this exact name.
Set up basic permissions for stack set operations
The above template was created based on files published by AWS official.
IAM role for the target account
Resources:
ExecutionRole:
Type: AWS::IAM::Role
Properties:
RoleName: AWSCloudFormationStackSetExecutionRole
AssumeRolePolicyDocument:
Version: 2012-10-17
Statement:
- Effect: Allow
Principal:
AWS:
- !Ref AWS::AccountId
Action:
- sts:AssumeRole
Path: /
ManagedPolicyArns:
- !Sub "arn:${AWS::Partition}:iam::aws:policy/AdministratorAccess"
Code language: YAML (yaml)
The second is the IAM role for the target account.
In each target account, create a service role named AWSCloudFormationStackSetExecutionRole that trusts the administrator account. The role must have this exact name.
Set up basic permissions for stack set operations
This time, since we are creating the stack in our own account, the target account will also be ourselves.
So we will create an IAM role for the target as well as an IAM role for the admin account.
The above template was created based on files published by AWS official.
The TemplateURL property specifies the template file used to generate each stack.
The template file is placed in an S3 bucket and its URL is specified.
The URL should be in virtual hosting format.
https://[bucket-name].s3.[region].amazonaws.com/[template-file]
In this case, we will specify a template file containing the Lambda functions described below.
(Reference) Lambda function
Resources:
Function:
Type: AWS::Lambda::Function
Properties:
Environment:
Variables:
REGION: !Ref AWS::Region
MEMORY: !Ref Memory
Code:
ZipFile: |
import json
import os
region = os.environ['REGION']
memory = os.environ['MEMORY']
def lambda_handler(event, context):
data = {
'region': region,
'memory': memory
}
return {
'statusCode': 200,
'body': json.dumps(data, indent=2)
}
FunctionName: !Sub "${Prefix}-function"
Handler: !Ref Handler
MemorySize: !Ref Memory
Runtime: !Ref Runtime
Role: !GetAtt FunctionRole.Arn
Code language: YAML (yaml)
This is a Lambda function generated by StackSets for each region.
This is a simple one that returns region information and amount of memory.
Architecting
Use CloudFormation to build this environment and check its actual behavior.
Create CloudFormation stacks and check the resources in the stack
Create CloudFormation stacks.
For information on how to create stacks and check each stack, please see the following page.
In this case, to generate a named IAM resource, the command is as follows.
aws cloudformation create-stack \
--stack-name fa-135 \
--template-url https://[bucket-name].s3.ap-northeast-1.amazonaws.com/[path]/fa-135.yaml \
--capabilities CAPABILITY_NAMED_IAM
Code language: YAML (yaml)
After reviewing the resources in each stack, information on the main resources created in this case is as follows
- StackSets:fa-135
Check each resource from the AWS Management Console.
Check StackSets.
StackSet has been successfully created.
Check the stack instance.
Indeed, we can see that stacks have been generated in two regions.
Check the default parameters.
Four parameters are passed.
The key point is that the memory value is “128”.
Operation Check
Now that we are ready, we check each generated stack.
Stack 1
First, check the stack generated in ap-northeast-1.
You can see that Lambda functions and other functions are generated in the stack.
Also, looking at the parameters, we can see that the values we just checked in StackSets are passed.
Check the created Lambda function.
The function is created as specified in the template file. The amount of memory is indeed 128 (MB).
Execute this function.
Successfully executed.
The function was created in region ap-northeast-1 with 128 MB of memory.
Stack 2
Next, check the stack generated on us-east-1.
You can see that Lambda functions and other functions are generated in the stack.
Also, looking at the parameter, the amount of memory is “256”.
This is because we have overwritten the parameter value only for the us-east-1 region.
Check the created Lambda function.
The function code is as specified in the template file.
But the amount of memory is 256 (MB).
You can see that the memory parameter was indeed overwritten.
Execute this function.
Successfully executed.
Thus, a function was generated in region us-east-1 with 256 MB of memory.
Summary
As an introduction to StackSets, I used StackSets to deploy resources to two regions of my account.