Invoke Lambda functions periodically
EventBridge allows you to set up recurring actions.
In this case, we will invoke Lambda functions periodically.
Environment
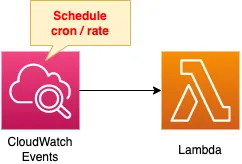
Create Lambda functions.
Set up scheduled expressions in EventBridge to invoke the functions periodically.
CloudFormation template files
Build the above configuration with CloudFormation.
The CloudFormation templates are located at the following URL
https://github.com/awstut-an-r/awstut-soa/tree/main/03/003
Explanation of key points of the template files
Lambda function
Resources:
Function1:
Type: AWS::Lambda::Function
Properties:
Code:
ZipFile: |
import datetime
def lambda_handler(event, context):
now = datetime.datetime.now()
now_str = now.strftime('%Y-%m-%d %H:%M:%S')
print(now_str)
FunctionName: !Sub "${Prefix}-function1"
Handler: !Ref Handler
Role: !GetAtt FunctionRole.Arn
Runtime: !Ref Runtime
Code language: YAML (yaml)
Create a simple Lambda function to validate EventBridge’s scheduling expressions.
In this case, we will describe the code to be executed inline.
For more information on how to create a Lambda function in CloudFormation, please check the following page
The code will retrieve the current date and time and output it.
We will prepare another similar function.
EventBridge
Rule
Resources:
Rule1:
Type: AWS::Events::Rule
Properties:
Name: !Sub "${Prefix}-EventsRule1"
ScheduleExpression: rate(1 minute)
State: ENABLED
Targets:
- Arn: !Ref FunctionArn1
Id: !Ref Function1
Rule2:
Type: AWS::Events::Rule
Properties:
Name: !Sub "${Prefix}-EventsRule2"
ScheduleExpression: cron(* * * * ? *)
State: ENABLED
Targets:
- Arn: !Ref FunctionArn2
Id: !Ref Function2
Code language: YAML (yaml)
The ScheduleExpression property specifies when the Lambda function will be invoked.
In this case, we will set the function to invoke every minute.
There are two types of notation.
The first is a rate expression.
Please refer to the following page for detailed description.
https://docs.aws.amazon.com/AmazonCloudWatch/latest/events/ScheduledEvents.html#RateExpressions
The second is a cron expression.
For details, please refer to the following page.
https://docs.aws.amazon.com/AmazonCloudWatch/latest/events/ScheduledEvents.html#CronExpressions
Specify the target resources to be executed with the Targets property.
In this case, Lambda functions are the target, so specify the ARNs of these functions.
Resource based policy
EventsRulePermission1:
Type: AWS::Lambda::Permission
Properties:
FunctionName: !Ref Function1
Action: lambda:InvokeFunction
Principal: events.amazonaws.com
SourceArn: !GetAtt Rule1.Arn
Code language: YAML (yaml)
When you use an EventBridge scheduled expression to invoke a Lambda function on a regular basis, the EventBridge invoke the Lambda function.
Therefore, you need to give the EventBridge rule the necessary permissions to invoke the function.
Create a resource-based policy and authorize the EventBridge rule to invoke the Lambda function (lambda:InvokeFunction).
There are two main ways to authorize EventBridge: creating a resource-based policy or creating an IAM role and associating it with a rule. In this case, we chose the former.
This is due to the following specifications for EventBridge and Lambda functions.
For Lambda, Amazon SNS, Amazon SQS, and Amazon CloudWatch Logs resources, EventBridge uses resource-based policies.
Using resource-based policies for Amazon EventBridge
Architecting
Use CloudFormation to build this environment and check the actual behavior.
Create CloudFormation stacks and check resources in stacks
Create a CloudFormation stacks.
For information on how to create a stack and check each stack, please refer to the following page
After checking the resources in each stack, information on the main resources created this time is as follows
- Lambda function 1: soa-03-003-function1
- Lambda function 2: soa-03-003-function2
- EventBridge rule 1: soa-03-003-EventsRule1
- EventBridge rule 2: soa-03-003-EventsRule2
Check the SSM status from the AWS Management Console as well.
Check the EventBridge rules.
First, let’s check the first rule.
We can see that the schedule is set with a rate expression.
Looking at the target resource, we can also see that the first Lambda function is specified.
Next, check the second rule.
We can see that the schedule is set up with a cron expression.
We also see that the second Lambda function is specified as the target.
Check Action
Now that everything is ready, let’s check the execution logs of the two functions.
First, let’s check the log of the first function scheduled with the rate expression.
We can see that date/time data is written every minute.
Indeed, the rate expression indicates that a Lambda function is invoked every minute.
Next, check the log of the second function scheduled by the cron expression.
You can see that the date and time data is also written every minute.
It is true that the cron expression also shows that a Lambda function is invoked every minute.
Summary
We have confirmed how to invoke Lambda functions periodically by using EventBridge’s scheduled expressions (rate/cron expressions).